I recently found a fun programming challenge that was issued by a well known hotel chain. The task is to recreate the portion of a website where the user selects which rooms they would like to rent as well as the number of occupants per room.
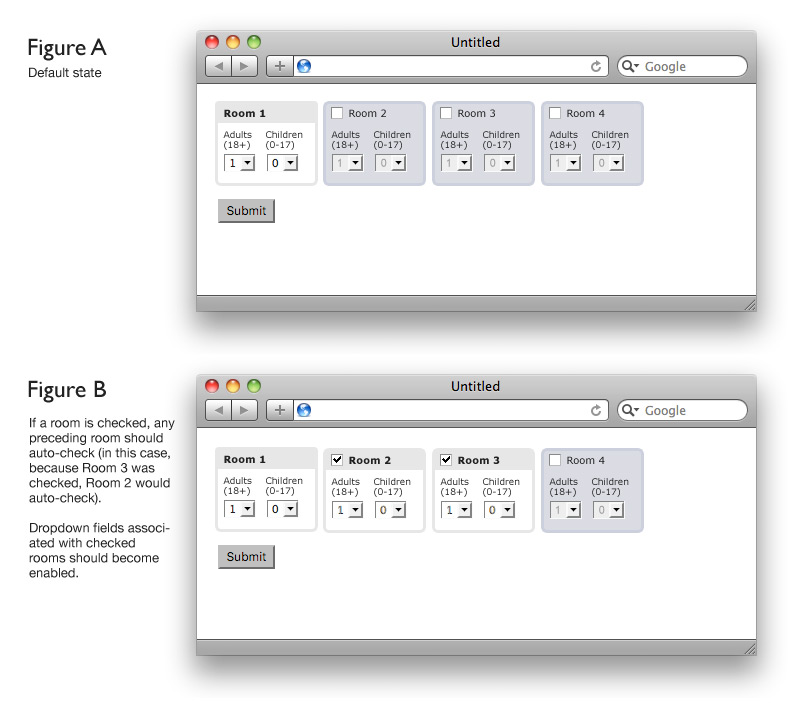
In technical terms, the HTML and CSS are really easy to recreate using flexbox and the functionality can be achieved with a bit of javaScript. I've added a codepen here if you want to see what that looks like:
And below is the JavaScript code with comments. It's been a while since I've been able to write a bit of vanilla JS. Most of my day to day work makes use of React, Angular, or Node which make it a bit quicker to do some things. Honestly, there's nothing wrong with doing things without a framework for most smaller websites. In my opinion, being able to manipulate the DOM using just the language itself give you a good foundation for understanding some of the abstractions that make your life easier when using modern front end frameworks.
//getting arrays of the checkBoxes and selects
const checkBoxes = document.querySelectorAll( '.check' );
const selects = document.querySelectorAll( '.dropdown' );
//setting the selects to be disabled by default
for ( let i = 0; i < selects.length; i++ ) {
selects[ i ].disabled = true;
}
//loop over the checkboxes and add an event listener on each one
//if one is checked, I check all previous boxes
//if one is unchecked, all rooms that come after it should be unchecked.
checkBoxes.forEach( ( checkInput, idx, checks ) => {
checkInput.addEventListener( 'click', function () {
if ( checkInput.checked ) {
for ( let i = idx; i >= 0; i-- ) {
checks[ i ].checked = true;
if ( i === 0 ) {
selects[ 0 ].disabled = false;
selects[ 1 ].disabled = false;
} else if ( i === 1 ) {
selects[ 2 ].disabled = false;
selects[ 3 ].disabled = false;
} else {
selects[ 4 ].disabled = false;
selects[ 5 ].disabled = false;
}
}
} else if ( !checkInput.checked ) {
for ( let i = idx; i < checks.length; i++ ) {
checks[ i ].checked = false;
if ( i === 0 ) {
selects[ 0 ].disabled = true;
selects[ 1 ].disabled = true;
} else if ( i === 1 ) {
selects[ 2 ].disabled = true;
selects[ 3 ].disabled = true;
} else {
selects[ 4 ].disabled = true;
selects[ 5 ].disabled = true;
}
}
}
} );
} );